Posted in
Windows Powershell,
Windows Server |
No Comment | 3,082 views | 30/06/2014 17:32
You can use following script to get Port and Fabric WWN information from FCinfo via PowerShell.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
| # Get FCInfo Details
$FcToolCmd = "C:\StorageTools\fcinfo\fcinfo.exe /details"
# Create WWN Array
$WWNArray = @()
# Create Hash Table
$WWNInformation = @{}
# Port WWN Information
$FabricDetails = Invoke-Expression $FcToolCmd | Where {$_ -match "port_wwn"}
foreach ($FabricInfo in $FabricDetails)
{
# Get Port WWN
$FabricRegex = $FabricInfo -match "\b\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\b"
$PortWWN = $Matches[0]
# Add Hash Table
$WWNInformation."$PortWWN" = "NULL"
# Add WWN Array
$WWNArray += $PortWWN
}
# Fabric Index
$FabricIndex = 0;
# Fabric WWN Information
$FabricDetails = Invoke-Expression $FcToolCmd | Where {$_ -match "fabric"}
foreach ($FabricInfo in $FabricDetails)
{
# Get Fabric WWN
$FabricRegex = $FabricInfo -match "\b\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\b"
$FabricWWN = $Matches[0]
# Get Port WWN
$PortWWN = $WWNArray[$FabricIndex]
# Add Hash Table
$WWNInformation."$PortWWN" = "$FabricWWN"
# Update Fabric Index
$FabricIndex++
}
# Output Results
$WWNInformation |
# Get FCInfo Details
$FcToolCmd = "C:\StorageTools\fcinfo\fcinfo.exe /details"
# Create WWN Array
$WWNArray = @()
# Create Hash Table
$WWNInformation = @{}
# Port WWN Information
$FabricDetails = Invoke-Expression $FcToolCmd | Where {$_ -match "port_wwn"}
foreach ($FabricInfo in $FabricDetails)
{
# Get Port WWN
$FabricRegex = $FabricInfo -match "\b\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\b"
$PortWWN = $Matches[0]
# Add Hash Table
$WWNInformation."$PortWWN" = "NULL"
# Add WWN Array
$WWNArray += $PortWWN
}
# Fabric Index
$FabricIndex = 0;
# Fabric WWN Information
$FabricDetails = Invoke-Expression $FcToolCmd | Where {$_ -match "fabric"}
foreach ($FabricInfo in $FabricDetails)
{
# Get Fabric WWN
$FabricRegex = $FabricInfo -match "\b\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\:\S{2}\b"
$FabricWWN = $Matches[0]
# Get Port WWN
$PortWWN = $WWNArray[$FabricIndex]
# Add Hash Table
$WWNInformation."$PortWWN" = "$FabricWWN"
# Update Fabric Index
$FabricIndex++
}
# Output Results
$WWNInformation
That will give you a hashtable as a result, so you can see details.
Posted in
Windows Powershell,
Windows Server |
No Comment | 38,084 views | 27/06/2014 09:55
Following scripts require EMC Storage Integrator (ESI) PowerShell Toolkit (ESIPSToolkit).
Getting EMC Storage Snapshot Pools:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| Import-Module ESIPSToolkit
function Get-EMCStorageSnapPools {
$Storages = Get-EMCStorageSystem
foreach ($Storage in $Storages) {
$GlobalID = $Storage.GlobalID
$Snappool = Get-EMCSnapshotPool | Where StorageSystemGlobalId -eq $GlobalID
$Properties = New-Object Psobject
$Properties | Add-Member Noteproperty StorageName $Storage.UserFriendlyName
$Properties | Add-Member Noteproperty AvailableCapacity $Snappool.AvailableCapacity
$Properties | Add-Member Noteproperty UserCapacity $Snappool.UserCapacity
Write-Output $Properties
}
}
Get-EMCStorageSnapPools |
Import-Module ESIPSToolkit
function Get-EMCStorageSnapPools {
$Storages = Get-EMCStorageSystem
foreach ($Storage in $Storages) {
$GlobalID = $Storage.GlobalID
$Snappool = Get-EMCSnapshotPool | Where StorageSystemGlobalId -eq $GlobalID
$Properties = New-Object Psobject
$Properties | Add-Member Noteproperty StorageName $Storage.UserFriendlyName
$Properties | Add-Member Noteproperty AvailableCapacity $Snappool.AvailableCapacity
$Properties | Add-Member Noteproperty UserCapacity $Snappool.UserCapacity
Write-Output $Properties
}
}
Get-EMCStorageSnapPools
Getting Storage List:
1
2
3
| Import-Module ESIPSToolkit
$Storage = Get-EMCStorageSystem
$Storage.UserFriendlyName |
Import-Module ESIPSToolkit
$Storage = Get-EMCStorageSystem
$Storage.UserFriendlyName
Getting Storage Thin Pools:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
| Import-Module ESIPSToolkit
function Get-EMCStorageThinPools {
$Storages = Get-EMCStorageSystem
foreach ($Storage in $Storages) {
$GlobalID = $Storage.GlobalID
$Thinpool = Get-EMCStoragePool | Where SupportsThinProvisioning -eq "True" | where StorageSystemGlobalId -eq $GlobalID
foreach ($Pool in $Thinpool) {
$Properties = New-Object Psobject
$Properties | Add-Member Noteproperty StorageName $storage.UserFriendlyName
$Properties | Add-Member Noteproperty PoolName $pool.Name
$Properties | Add-Member Noteproperty TotalCapacity $pool.TotalCapacity.ValueGB
$Properties | Add-Member Noteproperty AvailableCapacity $pool.AvailableCapacity.ValueGB
$Properties | Add-Member Noteproperty SubscribedCapacity $pool.SubscribedCapacity.ValueGB
Write-Output $Properties
}
}
}
Get-EMCStorageThinPools |
Import-Module ESIPSToolkit
function Get-EMCStorageThinPools {
$Storages = Get-EMCStorageSystem
foreach ($Storage in $Storages) {
$GlobalID = $Storage.GlobalID
$Thinpool = Get-EMCStoragePool | Where SupportsThinProvisioning -eq "True" | where StorageSystemGlobalId -eq $GlobalID
foreach ($Pool in $Thinpool) {
$Properties = New-Object Psobject
$Properties | Add-Member Noteproperty StorageName $storage.UserFriendlyName
$Properties | Add-Member Noteproperty PoolName $pool.Name
$Properties | Add-Member Noteproperty TotalCapacity $pool.TotalCapacity.ValueGB
$Properties | Add-Member Noteproperty AvailableCapacity $pool.AvailableCapacity.ValueGB
$Properties | Add-Member Noteproperty SubscribedCapacity $pool.SubscribedCapacity.ValueGB
Write-Output $Properties
}
}
}
Get-EMCStorageThinPools
Registering Host:
1
2
3
| $StorageArray = Get-EMCStorageSystem "CX4_1"
$HostRegistration = "C0:03:FF:00:00:FF:FF:00:C0:03:FF:65:4D:A6:00:00","C0:03:FF:00:00:FF:FF:00:C0:03:FF:37:25:4E:00:16"
$RegisterHost = New-EMCStorageRegisteredHost -StorageSystem $StorageArray -HostName "Server01" -IpAddress "192.168.0.114" -HostBusAdapterIds $HostRegistration |
$StorageArray = Get-EMCStorageSystem "CX4_1"
$HostRegistration = "C0:03:FF:00:00:FF:FF:00:C0:03:FF:65:4D:A6:00:00","C0:03:FF:00:00:FF:FF:00:C0:03:FF:37:25:4E:00:16"
$RegisterHost = New-EMCStorageRegisteredHost -StorageSystem $StorageArray -HostName "Server01" -IpAddress "192.168.0.114" -HostBusAdapterIds $HostRegistration
To be continued.. :)
Posted in
Windows Server |
No Comment | 10,666 views | 26/06/2014 11:16
You can apply this Bios settings to HP Proliant DL980 and HP Proliant DL580 for your Microsoft SQL Servers.

You can review full notes from “Best Practices for Tuning Microsoft SQL Server on the HP Proliant DL980 Server”:
Whitepaper is written for DL980 but HP confirms that Bios settings also applies for DL580.
Thanks to Bunyamin Topan for sharing this information :)
Posted in
Virtual Machine Manager,
Windows Powershell,
Windows Server |
No Comment | 2,037 views | 24/06/2014 09:42
You can update all SCVMM agents with following script:
1
2
3
4
5
6
7
| $Credential = Get-SCRunAsAccount -Name "Domain_Account"
$HyperVHosts = Get-SCVMMManagedComputer
foreach ($HyperVHost in $HyperVHosts)
{
$ManagedComputer = Get-SCVMMManagedComputer -ComputerName $HyperVHost
Update-SCVMMManagedComputer -Credential $Credential -VMMManagedComputer $HyperVHost
} |
$Credential = Get-SCRunAsAccount -Name "Domain_Account"
$HyperVHosts = Get-SCVMMManagedComputer
foreach ($HyperVHost in $HyperVHosts)
{
$ManagedComputer = Get-SCVMMManagedComputer -ComputerName $HyperVHost
Update-SCVMMManagedComputer -Credential $Credential -VMMManagedComputer $HyperVHost
}
That will update all Hyper-V hosts agents as soon as possible.
Posted in
Windows Powershell,
Windows Server |
3 Comments | 1,989 views | 23/06/2014 11:44
This is third version of Hyper-V Cluster Memory Reports.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
| $Clusters = Get-Content Clusters.txt
foreach ($Cluster in $Clusters)
{
[int]$TotalFreeMemory = 0;
[int]$TotalMemory = 0;
[int]$TotalUsedMemory = 0;
[int]$NodeCount = 0;
$ClusterNodes = Get-Cluster $Cluster | Get-ClusterNode
foreach ($ClusterNode in $ClusterNodes)
{
[int]$FreeMemory = [math]::round(((Get-WmiObject -ComputerName $ClusterNode -Class Win32_OperatingSystem).FreePhysicalMemory / 1MB), 0) - ([math]::round(((Get-WmiObject -ComputerName $ClusterNode -Class Win32_OperatingSystem).TotalVisibleMemorySize / 1MB), 0)*10/100)
[int]$TotalFreeMemory = [int]$TotalFreeMemory + [int]$FreeMemory
[int]$NodeMemory = [math]::round(((Get-WmiObject -ComputerName $ClusterNode -Class Win32_OperatingSystem).TotalVisibleMemorySize / 1MB), 0)
[int]$TotalMemory = [int]$TotalMemory + [int]$NodeMemory
[int]$UsedMemory = [int]$NodeMemory - [int]$FreeMemory
[int]$TotalUsedMemory = [int]$TotalUsedMemory + [int]$UsedMemory;
[int]$NodeCount = [int]$NodeCount + 1
}
[int]$TotalAvailableMemory = [int]$TotalFreeMemory - [int]$NodeMemory - ([int]$NodeMemory*10/100)
Write-Host "Cluster: $Cluster"
Write-Host "Total Memory: $TotalMemory"
Write-Host "Total Free Memory: $TotalFreeMemory"
Write-Host "Total Used Memory: $TotalUsedMemory"
Write-Host "Total Available Memory: $TotalAvailableMemory"
Write-Host "Node Count: $NodeCount"
Write-Host " "
$Value = $Cluster + ";" + $TotalMemory + ";" + $TotalFreeMemory + ";" + $TotalUsedMemory + ";" + $TotalAvailableMemory + ";" + $NodeCount + ";" + $NodeMemory
Add-Content -Value $Value -Path Memory.txt
} |
$Clusters = Get-Content Clusters.txt
foreach ($Cluster in $Clusters)
{
[int]$TotalFreeMemory = 0;
[int]$TotalMemory = 0;
[int]$TotalUsedMemory = 0;
[int]$NodeCount = 0;
$ClusterNodes = Get-Cluster $Cluster | Get-ClusterNode
foreach ($ClusterNode in $ClusterNodes)
{
[int]$FreeMemory = [math]::round(((Get-WmiObject -ComputerName $ClusterNode -Class Win32_OperatingSystem).FreePhysicalMemory / 1MB), 0) - ([math]::round(((Get-WmiObject -ComputerName $ClusterNode -Class Win32_OperatingSystem).TotalVisibleMemorySize / 1MB), 0)*10/100)
[int]$TotalFreeMemory = [int]$TotalFreeMemory + [int]$FreeMemory
[int]$NodeMemory = [math]::round(((Get-WmiObject -ComputerName $ClusterNode -Class Win32_OperatingSystem).TotalVisibleMemorySize / 1MB), 0)
[int]$TotalMemory = [int]$TotalMemory + [int]$NodeMemory
[int]$UsedMemory = [int]$NodeMemory - [int]$FreeMemory
[int]$TotalUsedMemory = [int]$TotalUsedMemory + [int]$UsedMemory;
[int]$NodeCount = [int]$NodeCount + 1
}
[int]$TotalAvailableMemory = [int]$TotalFreeMemory - [int]$NodeMemory - ([int]$NodeMemory*10/100)
Write-Host "Cluster: $Cluster"
Write-Host "Total Memory: $TotalMemory"
Write-Host "Total Free Memory: $TotalFreeMemory"
Write-Host "Total Used Memory: $TotalUsedMemory"
Write-Host "Total Available Memory: $TotalAvailableMemory"
Write-Host "Node Count: $NodeCount"
Write-Host " "
$Value = $Cluster + ";" + $TotalMemory + ";" + $TotalFreeMemory + ";" + $TotalUsedMemory + ";" + $TotalAvailableMemory + ";" + $NodeCount + ";" + $NodeMemory
Add-Content -Value $Value -Path Memory.txt
}
You should add your clusters into Clusters.txt file.
Posted in
Windows Powershell,
Windows Server |
No Comment | 13,349 views | 22/06/2014 17:35
My name is Yusuf Ozturk. I’m a PowerShell MVP and a system engineer at the Cloud team of a private bank where we have biggest Hyper-V production environment in Turkey. Managing large virtualization environment could be a headache if you don’t do your growing plans well or if you are growing quickly than you are expected. Patching Microsoft Updates to your Hyper-V Clusters could be problematic if you face with following issues:
1. Don’t have enough memory
2. Don’t have enough disk space
That means, your cluster is over-committed..
Also this issues could increase your pain in this scenario:
1. VMs with large memory (like 64GB memory or more)
2. VMs with vHBA
3. VMs with Passthrough Disks
Because you should do a good planing if you have large VMs in a over-committed environment because migrating that VMs won’t be an easy job than you think. Also misconfigured SAN switches, WWN configurations etc could be a problem for VMs with vHBa. Also drivers and firmwares could cause some issues for vHBA. As my experience, best way for migrating a VM with vHBA is keeping that offline. Otherwise you can lose disk connectivity anyway and it could damage your system, even you can lose data. So using clustered vHBA VMs can increase your uptime but also prevents data lose.
For the Passthrough disks, Hyper-V always get some issues with PT disks, couldn’t handle it well. So Microsoft doesn’t recommend it anymore maybe they will stop support with vNext, who knows..
So with this scenarios, current built-in Cluster-Aware Updating (CAU) has some problems:
1. CAU doesn’t know your VMs with vHBA or PT, because Cluster is handling them..
2. CAU doesn’t know your memory issue
3. CAU doesn’t know your disk issue
4. CAU doesn’t know if VM has a large memory
That’s why you can see that, Cluster tries to send a VM with 64 GB memory into a Hyper-V host with 32 GB free memory. Because Cluster only checks free memory in Cluster nodes. So i re-imagined CAU and wrote my own CAU process. What I do in my scenarion:
1. I can suspend VMs with vHBA and PT disk unless Cluster Node is online. (This is my choice, you can always use Live migrations)
2. I know which Cluster node has largest free memory. But what I know more is, which VM has largest memory as well. So I start with largest memory footprint VM, migrate it to best Cluster node. After that I can start migrating low memory VMs into that Host because there are still some memory for VMs like 2 GB, 4 GB etc..
3. I don’t leave migrations to Cluster Management. I do my own migrations. So that will give me my own control mechanism and that will give me flexibility. I can set timeout for VM migrations, so if a VM could not migrate in timely fashion, I can migrate it offline..
This is my own steps. You can always edit my script for your own requirements. Now lets see my new CAU script:
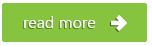
Posted in
Windows Powershell,
Windows Server |
1 Comment | 2,733 views | 20/06/2014 22:51
You may want to check available Microsoft Updates on remote servers. This script will give you that info.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
| Function Get-MicrosoftUpdate {
<#
.SYNOPSIS
Function to get available Microsoft Updates on Windows Servers.
.DESCRIPTION
If you have large number of Windows Servers, Microsoft Updates may not be an easy job.
This script could get available Microsoft Updates from remote servers by using PowerShell.
.PARAMETER WhatIf
Display what would happen if you would run the function with given parameters.
.PARAMETER Confirm
Prompts for confirmation for each operation. Allow user to specify Yes/No to all option to stop prompting.
.EXAMPLE
Get-MicrosoftUpdate
.EXAMPLE
Get-MicrosoftUpdate -ComputerName Server01
.EXAMPLE
Get-MicrosoftUpdate -ComputerName Server01 -SuppressMode
.INPUTS
None
.OUTPUTS
None
.NOTES
Author: Yusuf Ozturk
Website: http://www.yusufozturk.info
Email: ysfozy@gmail.com
Date created: 19-June-2014
Last modified: 22-June-2014
Version: 1.2
.LINK
http://www.yusufozturk.info
http://twitter.com/yusufozturk
#>
[CmdletBinding(SupportsShouldProcess = $true)]
param (
# ComputerName
[Parameter(
Mandatory = $false,
HelpMessage = 'Computer Name')]
$ComputerName = "localhost",
# Suppress Mode
[Parameter(
Mandatory = $false,
HelpMessage = 'Suppress Mode')]
[switch]$SuppressMode = $false,
# Debug Mode
[Parameter(
Mandatory = $false,
HelpMessage = 'Debug Mode')]
[switch]$DebugMode = $false
)
# Enable Debug Mode
if ($DebugMode)
{
# Set Error Action Preference
$ErrorActionPreference = "Stop"
# Set Debug Preference
$DebugPreference = "Continue"
}
else
{
# Set Error Action Preference
$ErrorActionPreference = "silentlycontinue"
}
if ($ComputerName -ne "localhost")
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Getting Microsoft Update information from $ComputerName.." -ForegroundColor Cyan
Write-Host "Please wait.." -ForegroundColor Gray
Write-Host " "
}
# Invoke Script on Remote Computer
$InvokeCommand = Invoke-Command -ComputerName $ComputerName -ArgumentList $SuppressMode, $DebugMode -ScriptBlock {
# Define Parameters
param ($SuppressMode, $DebugMode)
# Create Microsoft Update Session
$UpdateSession = New-Object -ComObject "Microsoft.Update.Session"
# Search Microsoft Updates
$UpdateSearcher = $UpdateSession.CreateUpdateSearcher()
$SearchResult = $UpdateSearcher.Search("IsInstalled=0 and IsHidden=0")
# Create Microsoft Update Install Queue
$InstallQueue = New-Object -ComObject "Microsoft.Update.UpdateColl"
for ($i = 0; $i -lt $SearchResult.Updates.Count; $i++)
{
# Get Current Microsoft Update
$Update = $SearchResult.Updates.Item($i)
# Get Microsoft Update Description
$UpdateDescription = $Update.Title
# Microsoft Update KB Pattern
$KBPattern = "KB\d{1,20}"
# Search for Microsoft Update KB
$RegexSearch = $UpdateDescription -match $KBPattern
# Get Microsoft Update KB
$UpdateKB = $Null;
$UpdateKB = $Matches[0]
if (!$SuppressMode)
{
# Informational Output
Write-Host "-------------------------------------------------"
Write-Host " "
Write-Host "$UpdateKB is available to install.." -ForegroundColor Cyan
Write-Host "Description: $UpdateDescription" -ForegroundColor Gray
Write-Host " "
}
# Add to Install Queue
$InstallQueue.Add($Update) | Out-Null
# Reboot Behaviour
if ($Update.InstallationBehavior.RebootBehavior -gt 0)
{
# Set Reboot Status
$RebootRequired = $True
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: $UpdateKB requires reboot." -ForegroundColor Yellow
Write-Host " "
Write-Host " "
}
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "$UpdateKB doesn't require reboot." -ForegroundColor Green
Write-Host " "
Write-Host " "
}
}
}
if ($InstallQueue.Count -eq 0)
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: There are no Microsoft Updates available" -ForegroundColor Yellow
}
# Update Status
$UpdateStatus = "False"
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Available Microsoft Updates: $i" -ForegroundColor Green
}
# Update Status
$UpdateStatus = "True"
}
# Output Update Status
$UpdateStatus
}
if ($SuppressMode)
{
# Output Invoke Command Results
$InvokeCommand
}
}
else
{
# Create Microsoft Update Session
$UpdateSession = New-Object -ComObject "Microsoft.Update.Session"
# Search Microsoft Updates
$UpdateSearcher = $UpdateSession.CreateUpdateSearcher()
$SearchResult = $UpdateSearcher.Search("IsInstalled=0 and IsHidden=0")
# Create Microsoft Update Install Queue
$InstallQueue = New-Object -ComObject "Microsoft.Update.UpdateColl"
for ($i = 0; $i -lt $SearchResult.Updates.Count; $i++)
{
# Get Current Microsoft Update
$Update = $SearchResult.Updates.Item($i)
# Get Microsoft Update Description
$UpdateDescription = $Update.Title
# Microsoft Update KB Pattern
$KBPattern = "KB\d{1,20}"
# Search for Microsoft Update KB
$RegexSearch = $UpdateDescription -match $KBPattern
# Get Microsoft Update KB
$UpdateKB = $Null;
$UpdateKB = $Matches[0]
if (!$SuppressMode)
{
# Informational Output
Write-Host "-------------------------------------------------"
Write-Host " "
Write-Host "$UpdateKB is available to install.." -ForegroundColor Cyan
Write-Host "Description: $UpdateDescription" -ForegroundColor Gray
Write-Host " "
}
# Add to Install Queue
$InstallQueue.Add($Update) | Out-Null
# Reboot Behaviour
if ($Update.InstallationBehavior.RebootBehavior -gt 0)
{
# Set Reboot Status
$RebootRequired = $True
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: $UpdateKB requires reboot." -ForegroundColor Yellow
Write-Host " "
Write-Host " "
}
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "$UpdateKB doesn't require reboot." -ForegroundColor Green
Write-Host " "
Write-Host " "
}
}
}
if ($InstallQueue.Count -eq 0)
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: There are no Microsoft Updates available" -ForegroundColor Yellow
}
# Update Status
$UpdateStatus = "False"
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Available Microsoft Updates: $i" -ForegroundColor Green
}
# Update Status
$UpdateStatus = "True"
}
if ($SuppressMode)
{
# Output Update Status
$UpdateStatus
}
}
} |
Function Get-MicrosoftUpdate {
<#
.SYNOPSIS
Function to get available Microsoft Updates on Windows Servers.
.DESCRIPTION
If you have large number of Windows Servers, Microsoft Updates may not be an easy job.
This script could get available Microsoft Updates from remote servers by using PowerShell.
.PARAMETER WhatIf
Display what would happen if you would run the function with given parameters.
.PARAMETER Confirm
Prompts for confirmation for each operation. Allow user to specify Yes/No to all option to stop prompting.
.EXAMPLE
Get-MicrosoftUpdate
.EXAMPLE
Get-MicrosoftUpdate -ComputerName Server01
.EXAMPLE
Get-MicrosoftUpdate -ComputerName Server01 -SuppressMode
.INPUTS
None
.OUTPUTS
None
.NOTES
Author: Yusuf Ozturk
Website: http://www.yusufozturk.info
Email: ysfozy@gmail.com
Date created: 19-June-2014
Last modified: 22-June-2014
Version: 1.2
.LINK
http://www.yusufozturk.info
http://twitter.com/yusufozturk
#>
[CmdletBinding(SupportsShouldProcess = $true)]
param (
# ComputerName
[Parameter(
Mandatory = $false,
HelpMessage = 'Computer Name')]
$ComputerName = "localhost",
# Suppress Mode
[Parameter(
Mandatory = $false,
HelpMessage = 'Suppress Mode')]
[switch]$SuppressMode = $false,
# Debug Mode
[Parameter(
Mandatory = $false,
HelpMessage = 'Debug Mode')]
[switch]$DebugMode = $false
)
# Enable Debug Mode
if ($DebugMode)
{
# Set Error Action Preference
$ErrorActionPreference = "Stop"
# Set Debug Preference
$DebugPreference = "Continue"
}
else
{
# Set Error Action Preference
$ErrorActionPreference = "silentlycontinue"
}
if ($ComputerName -ne "localhost")
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Getting Microsoft Update information from $ComputerName.." -ForegroundColor Cyan
Write-Host "Please wait.." -ForegroundColor Gray
Write-Host " "
}
# Invoke Script on Remote Computer
$InvokeCommand = Invoke-Command -ComputerName $ComputerName -ArgumentList $SuppressMode, $DebugMode -ScriptBlock {
# Define Parameters
param ($SuppressMode, $DebugMode)
# Create Microsoft Update Session
$UpdateSession = New-Object -ComObject "Microsoft.Update.Session"
# Search Microsoft Updates
$UpdateSearcher = $UpdateSession.CreateUpdateSearcher()
$SearchResult = $UpdateSearcher.Search("IsInstalled=0 and IsHidden=0")
# Create Microsoft Update Install Queue
$InstallQueue = New-Object -ComObject "Microsoft.Update.UpdateColl"
for ($i = 0; $i -lt $SearchResult.Updates.Count; $i++)
{
# Get Current Microsoft Update
$Update = $SearchResult.Updates.Item($i)
# Get Microsoft Update Description
$UpdateDescription = $Update.Title
# Microsoft Update KB Pattern
$KBPattern = "KB\d{1,20}"
# Search for Microsoft Update KB
$RegexSearch = $UpdateDescription -match $KBPattern
# Get Microsoft Update KB
$UpdateKB = $Null;
$UpdateKB = $Matches[0]
if (!$SuppressMode)
{
# Informational Output
Write-Host "-------------------------------------------------"
Write-Host " "
Write-Host "$UpdateKB is available to install.." -ForegroundColor Cyan
Write-Host "Description: $UpdateDescription" -ForegroundColor Gray
Write-Host " "
}
# Add to Install Queue
$InstallQueue.Add($Update) | Out-Null
# Reboot Behaviour
if ($Update.InstallationBehavior.RebootBehavior -gt 0)
{
# Set Reboot Status
$RebootRequired = $True
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: $UpdateKB requires reboot." -ForegroundColor Yellow
Write-Host " "
Write-Host " "
}
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "$UpdateKB doesn't require reboot." -ForegroundColor Green
Write-Host " "
Write-Host " "
}
}
}
if ($InstallQueue.Count -eq 0)
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: There are no Microsoft Updates available" -ForegroundColor Yellow
}
# Update Status
$UpdateStatus = "False"
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Available Microsoft Updates: $i" -ForegroundColor Green
}
# Update Status
$UpdateStatus = "True"
}
# Output Update Status
$UpdateStatus
}
if ($SuppressMode)
{
# Output Invoke Command Results
$InvokeCommand
}
}
else
{
# Create Microsoft Update Session
$UpdateSession = New-Object -ComObject "Microsoft.Update.Session"
# Search Microsoft Updates
$UpdateSearcher = $UpdateSession.CreateUpdateSearcher()
$SearchResult = $UpdateSearcher.Search("IsInstalled=0 and IsHidden=0")
# Create Microsoft Update Install Queue
$InstallQueue = New-Object -ComObject "Microsoft.Update.UpdateColl"
for ($i = 0; $i -lt $SearchResult.Updates.Count; $i++)
{
# Get Current Microsoft Update
$Update = $SearchResult.Updates.Item($i)
# Get Microsoft Update Description
$UpdateDescription = $Update.Title
# Microsoft Update KB Pattern
$KBPattern = "KB\d{1,20}"
# Search for Microsoft Update KB
$RegexSearch = $UpdateDescription -match $KBPattern
# Get Microsoft Update KB
$UpdateKB = $Null;
$UpdateKB = $Matches[0]
if (!$SuppressMode)
{
# Informational Output
Write-Host "-------------------------------------------------"
Write-Host " "
Write-Host "$UpdateKB is available to install.." -ForegroundColor Cyan
Write-Host "Description: $UpdateDescription" -ForegroundColor Gray
Write-Host " "
}
# Add to Install Queue
$InstallQueue.Add($Update) | Out-Null
# Reboot Behaviour
if ($Update.InstallationBehavior.RebootBehavior -gt 0)
{
# Set Reboot Status
$RebootRequired = $True
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: $UpdateKB requires reboot." -ForegroundColor Yellow
Write-Host " "
Write-Host " "
}
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "$UpdateKB doesn't require reboot." -ForegroundColor Green
Write-Host " "
Write-Host " "
}
}
}
if ($InstallQueue.Count -eq 0)
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Warning: There are no Microsoft Updates available" -ForegroundColor Yellow
}
# Update Status
$UpdateStatus = "False"
}
else
{
if (!$SuppressMode)
{
# Informational Output
Write-Host "Available Microsoft Updates: $i" -ForegroundColor Green
}
# Update Status
$UpdateStatus = "True"
}
if ($SuppressMode)
{
# Output Update Status
$UpdateStatus
}
}
}
So if you just type:
that will get Microsoft Updates on your local server.
If you want to get Microsoft Updates from remote server, then type like:
Get-MicrosoftUpdate -ComputerName "MyHyperVHost01" |
Get-MicrosoftUpdate -ComputerName "MyHyperVHost01"
That will give you Reboot requirements as well.