Categories
Sponsors
Archive
 Blogroll
Badges
Community
|
Posted in Windows Powershell, Windows Server | No Comment | 2,655 views | 30/01/2012 01:36
Check-PSSnapin allows you to check specific PowerShell Snapin on server.
I’m using this function in SetLinuxVM 3.0 to check SCVMM if it’s available on server.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
| # Check Powershell Snapin
# Author: Yusuf Ozturk
# http://www.yusufozturk.info
function Check-PSSnapin
{
param (
[Parameter(
Mandatory = $true,
HelpMessage = 'PowerShell Snapin Name. Example: Microsoft.SystemCenter.VirtualMachineManager')]
[string]$Name
)
$Success = "1";
$CheckSnapin = Get-PSSnapin -Name $Name -EA SilentlyContinue
if (!$CheckSnapin)
{
$AddSnapin = Add-PSSnapin -Name $Name -EA SilentlyContinue
$CheckSnapin = Get-PSSnapin -Name $Name -EA SilentlyContinue
if (!$CheckSnapin)
{
Write-Debug "$Name Snapin is not available."
}
else
{
Write-Debug "$Name Snapin is added."
$Success
}
}
else
{
Write-Debug "$Name Snapin is already loaded."
$Success
}
} |
# Check Powershell Snapin
# Author: Yusuf Ozturk
# http://www.yusufozturk.info
function Check-PSSnapin
{
param (
[Parameter(
Mandatory = $true,
HelpMessage = 'PowerShell Snapin Name. Example: Microsoft.SystemCenter.VirtualMachineManager')]
[string]$Name
)
$Success = "1";
$CheckSnapin = Get-PSSnapin -Name $Name -EA SilentlyContinue
if (!$CheckSnapin)
{
$AddSnapin = Add-PSSnapin -Name $Name -EA SilentlyContinue
$CheckSnapin = Get-PSSnapin -Name $Name -EA SilentlyContinue
if (!$CheckSnapin)
{
Write-Debug "$Name Snapin is not available."
}
else
{
Write-Debug "$Name Snapin is added."
$Success
}
}
else
{
Write-Debug "$Name Snapin is already loaded."
$Success
}
}
You can check PS Snapin like this:
Check-PSSnapin -Name "Microsoft.SystemCenter.VirtualMachineManager" |
Check-PSSnapin -Name "Microsoft.SystemCenter.VirtualMachineManager"
Name is mandatory, so you must give a name for that.
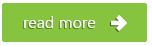
Posted in Windows Powershell, Windows Server | No Comment | 5,682 views | 30/01/2012 01:19
Check-WmiObject allows you to check Wmi provider/interface on given server.
I’m using this function in SetLinuxVM 3.0 to decide Hyper-V Manager.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
| # Check WMI Object
# Author: Yusuf Ozturk
# http://www.yusufozturk.info
function Check-WmiObject
{
param (
[Parameter(
Mandatory = $true,
HelpMessage = 'Wmi NameSpace. Example: root\virtualization')]
[string]$NameSpace,
[Parameter(
Mandatory = $false,
HelpMessage = 'Name of the Wmi Host. Example: Server01')]
[string]$WMIHost
)
$Success = "1";
if (!$WMIHost)
{
$CheckWmiObject = Get-WmiObject -Computer "." -Namespace "$NameSpace" -List -EA SilentlyContinue
if (!$CheckWmiObject)
{
Write-Debug "Could not contact with Wmi Provider."
}
else
{
Write-Debug "Wmi Provider is available."
$Success
}
}
else
{
$CheckWmiObject = Get-WmiObject -Computer "$WMIHost" -Namespace "$NameSpace" -List -EA SilentlyContinue
if (!$CheckWmiObject)
{
Write-Debug "Could not contact with Wmi Provider."
}
else
{
Write-Debug "Wmi Provider is available."
$Success
}
}
} |
# Check WMI Object
# Author: Yusuf Ozturk
# http://www.yusufozturk.info
function Check-WmiObject
{
param (
[Parameter(
Mandatory = $true,
HelpMessage = 'Wmi NameSpace. Example: root\virtualization')]
[string]$NameSpace,
[Parameter(
Mandatory = $false,
HelpMessage = 'Name of the Wmi Host. Example: Server01')]
[string]$WMIHost
)
$Success = "1";
if (!$WMIHost)
{
$CheckWmiObject = Get-WmiObject -Computer "." -Namespace "$NameSpace" -List -EA SilentlyContinue
if (!$CheckWmiObject)
{
Write-Debug "Could not contact with Wmi Provider."
}
else
{
Write-Debug "Wmi Provider is available."
$Success
}
}
else
{
$CheckWmiObject = Get-WmiObject -Computer "$WMIHost" -Namespace "$NameSpace" -List -EA SilentlyContinue
if (!$CheckWmiObject)
{
Write-Debug "Could not contact with Wmi Provider."
}
else
{
Write-Debug "Wmi Provider is available."
$Success
}
}
}
You can check Wmi Provider like this:
Check-WmiObject -NameSpace "root\virtualization" |
Check-WmiObject -NameSpace "root\virtualization"
If you want to query different wmi host:
Check-WmiObject -NameSpace "root\virtualization" -WmiHost "Server01" |
Check-WmiObject -NameSpace "root\virtualization" -WmiHost "Server01"
NameSpace is mandatory but you don’t need to type WmiHost if you query localhost.
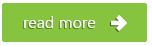
Posted in Linux Server, Virtual Machine Manager | 2 Comments | 9,857 views | 28/01/2012 20:07
You may need to uninstall Hyper-V Linux Integration Services v3.2 due to some reasons.
Let’s read documentation and see if we can.

After my first look, i don’t see any difference between x86 and x64.
Lets check it on VM console and see if they are correct.
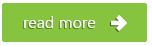
Posted in Windows Powershell | 3 Comments | 7,701 views | 16/01/2012 23:43
I wrote a calendar sync script for Outlook to sync calendars between Exchange accounts. If you need to sync your calendars, accounts should be in same Outlook profile. I didn’t figure out how to sync between different profiles yet, but will work on it.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
| Function Sync-OutlookCalendar
{
# Connect to Outlook
$Outlook = New-Object -com Outlook.Application
# Get Calendars
$Calendar1 = $Outlook.Session.folders.Item(1).Folders | where {$_.DefaultMessageClass -eq "IPM.Appointment"}
$Calendar2 = $Outlook.Session.folders.Item(2).Folders | where {$_.DefaultMessageClass -eq "IPM.Appointment"}
# Search Calendar Items
$Calendar1Items = $Calendar1.items | Where {$_.Start -gt (Get-Date)} | Select-Object -Property Start, End, Subject, Location, MeetingStatus
# Sync All: $Calendar1Items = $Calendar1.items | Select-Object -Property Start, End, Subject, Location, MeetingStatus
# Sync Calendar Items
Foreach ($Calendar1Item in $Calendar1Items)
{
$SearchDuplicate = $Calendar2.items | Where {$_.Start -eq $Calendar1Item.Start -and $_.End -eq $Calendar1Item.End -and $_.Subject -eq $Calendar1Item.Subject} | Select-Object -Property Start, End, Subject, Location, MeetingStatus
if ($SearchDuplicate -eq $null)
{
$Appointment = $Calendar2.Items.Add(1)
$Appointment.Start = $Calendar1Item.Start
$Appointment.End = $Calendar1Item.End
$Appointment.Subject = $Calendar1Item.Subject
$Appointment.Location = $Calendar1Item.Location
$Appointment.MeetingStatus = $Calendar1Item.MeetingStatus
$Appointment.Save()
}
else
{
Write-Host "Appointment is already exist"
}
}
} |
Function Sync-OutlookCalendar
{
# Connect to Outlook
$Outlook = New-Object -com Outlook.Application
# Get Calendars
$Calendar1 = $Outlook.Session.folders.Item(1).Folders | where {$_.DefaultMessageClass -eq "IPM.Appointment"}
$Calendar2 = $Outlook.Session.folders.Item(2).Folders | where {$_.DefaultMessageClass -eq "IPM.Appointment"}
# Search Calendar Items
$Calendar1Items = $Calendar1.items | Where {$_.Start -gt (Get-Date)} | Select-Object -Property Start, End, Subject, Location, MeetingStatus
# Sync All: $Calendar1Items = $Calendar1.items | Select-Object -Property Start, End, Subject, Location, MeetingStatus
# Sync Calendar Items
Foreach ($Calendar1Item in $Calendar1Items)
{
$SearchDuplicate = $Calendar2.items | Where {$_.Start -eq $Calendar1Item.Start -and $_.End -eq $Calendar1Item.End -and $_.Subject -eq $Calendar1Item.Subject} | Select-Object -Property Start, End, Subject, Location, MeetingStatus
if ($SearchDuplicate -eq $null)
{
$Appointment = $Calendar2.Items.Add(1)
$Appointment.Start = $Calendar1Item.Start
$Appointment.End = $Calendar1Item.End
$Appointment.Subject = $Calendar1Item.Subject
$Appointment.Location = $Calendar1Item.Location
$Appointment.MeetingStatus = $Calendar1Item.MeetingStatus
$Appointment.Save()
}
else
{
Write-Host "Appointment is already exist"
}
}
}
Every time you execute the script, it checks the duplicate entries. So you can’t sync if same appointment is also exist on other calendar. Also I thought to sync only new appointments, so there is a date checker too. You can change it if you want to sync all time appointments.
Posted in Hayattan | 4 Comments | 3,616 views | 15/01/2012 23:31
 |
For the effort of my last year, I’m honored by Microsoft as Most Valuable Professional (MVP) in PowerShell category. Thanks to Microsoft! I’m very glad and will work hard to be a MVP again. As a PowerShell MVP, started to work on new projects called PoSH Server, PoSH Panel, PoSH API and other secret projects :) PoSH Server is in preview, you can download it from http://www.poshserver.net and use it free. |
Posted in Hayattan | No Comment | 2,489 views | 15/01/2012 23:13
This is my 4th year on blogging. I started to post on this blog about 4 years ago and I still have same excitement and happiness as the first date of 2009. I want to give you some details about last year.
Total Visits: 135.000
Unique Visitors: 95.000
Page Views: 240.000
Top 5 Countries: Turkey, United States, United Kingdom, Germany, Netherlands
Last year’s results:
Total Visits: 75.000
Unique Visitors: 60.000
Page Views: 120.000
As you see, traffic increase is really good. I hope to see better results in 2013 :)
|